组合模式用于让客户端不再区分操作的是组合对象还是叶子对象,而是以一个统一的方式来操作。它的关键在于使用一个抽象的组件,来代表组合对象和叶子对象。这样客户就可以不用区分到底操作的是组合对象还是叶子对象了,只需要把他们全部当作组件对象进行统一操作就可以了。
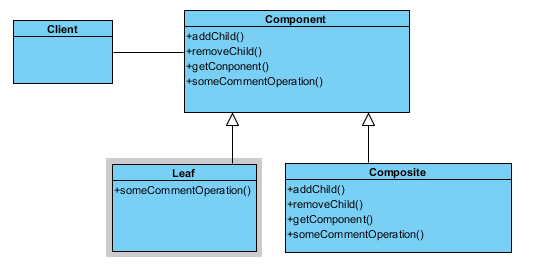
public abstract class Component { public String name = null; public Component(String name) { this.name = name; } public void addChild(Component component) { throw new UnsupportedOperationException(); } public void removeChild(Component component) { throw new UnsupportedOperationException(); } public Component getComponent(int index) { throw new UnsupportedOperationException(); } public abstract String getName(); }
|
import java.awt.List; import java.util.ArrayList; public class Compositive extends Component{ private ArrayList<Component> componentlist = new ArrayList<Component>(); public Compositive(String name) { super(name); } public String getName() { return super.name; } public void addChild(Component component) { if(component!= null) { componentlist.add(component); } } public void removeChild(Component component) { if(componentlist!= null && componentlist.contains(component)) { componentlist.remove(component); } } public Component getComponent(int index) { if(componentlist != null) { return componentlist.get(index); } return null; } }
|
public class Leaf extends Component { public Leaf(String name) { super(name); } public String getName() { return super.name; } }
|
public class Client { public static void main(String args[]) { Component component1 = new Compositive("组合1"); Component component2 = new Compositive("组合2"); Component component3 = new Compositive("组合3"); Component component4 = new Compositive("组合4"); Component component5 = new Compositive("组合5"); Component leaf1 = new Compositive("根节点1"); Component leaf2 = new Compositive("根节点2"); Component leaf3 = new Compositive("根节点3"); Component leaf4 = new Compositive("根节点4"); Component leaf5 = new Compositive("根节点5"); component1.addChild(component2); component1.addChild(component3); component1.addChild(component4); component1.addChild(component5); component2.addChild(leaf1); component2.addChild(leaf2); component3.addChild(leaf3); component4.addChild(leaf4); component4.addChild(leaf5); } }
|