原型模式:
原型模式是一种创建型模式,它一般用在需要快速创建大量相似的对象的情景下。
它与用new 创建对象的区别如下:
原型模式是一种内存二进制拷贝,所以比使用new 产生对象的方式来得快速高效。所以在需要重复地创建相似对象时可以考虑使用原型模式。比如需要在一个循环内创建对象,并且对象创建过程比较复杂或者循环次数很多的情况,使用原型模式不但可以简化创建过程,而且可以使系统的整体性能提高很多。
原型模式它创建对象的时候并不调用所创建对象的构造方法。这个是优点同时也是原型模式的缺点。
在使用原型模式的时候还需要注意的是浅拷贝和深拷贝
一般对于成员变量是基本数据类型,以及String类型的时候只需要使用浅拷贝即可,而对于那些成员变量包含数组,对象的类型,要注意使用深拷贝,否则那些成员变量是非基本变量的成员,在复制出来的对象中只是一个引用,这些引用指向的还是原对象,这样就会导致修改拷贝出的对象时,会对原对象产生干扰。
在原型模式中需要实现Cloneable接口。Cloneable它的作用只有一个,就是在运行时通知虚拟机可以安全地在实现了此接口的类上使用clone方法。在java虚拟机中,只有实现了这个接口的类才可以被拷贝,否则在运行时会抛出CloneNotSupportedException异常。
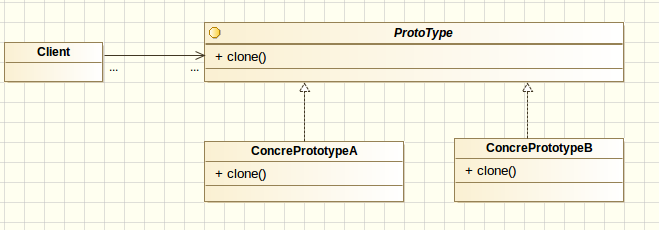
public class PersonInfo implements Cloneable{ private String name; private int age; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } protected Object clone() { PersonInfo info = null; try { info = (PersonInfo) super.clone(); } catch (CloneNotSupportedException e) { e.printStackTrace(); } return info; } }
|
public class CustomInfo implements Cloneable{
private long id = 0; private PersonInfo mPersonInfo = null; public CustomInfo() { System.out.println("调用构造方法"); } public long getId() { return id; } public void setId(long id) { this.id = id; } public PersonInfo getmPersonInfo() { return mPersonInfo; } public void setmPersonInfo(PersonInfo mPersonInfo) { this.mPersonInfo = mPersonInfo; } public Object clone() { CustomInfo info = null; try { info = (CustomInfo) super.clone(); info.setmPersonInfo((PersonInfo) this.mPersonInfo.clone()); } catch (CloneNotSupportedException e) { e.printStackTrace(); } return info; } public String toString() { return "id = "+id +" name = "+ mPersonInfo.getName()+ " age = " +mPersonInfo.getAge()+" hashCode = "+ this.hashCode(); } }
|
import java.util.Random; public class Client { public static void main(String args[]) { CustomInfo info = new CustomInfo(); info.setId(new Random().nextLong()); PersonInfo pinfo = new PersonInfo(); pinfo.setAge(new Random().nextInt(100)); pinfo.setName("Jimmy"); info.setmPersonInfo(pinfo); System.out.println(info.toString()); for(int i=0;i<10;i++) { CustomInfo cinfo = (CustomInfo) info.clone(); cinfo.setId(2222222222222l); System.out.println(cinfo.toString()); } System.out.println(info.toString()); } }
|